Detailed reference for the Fields object used in creating customized payment forms.
- fields.create()
- field.mount()
- field.on()
- Other methods
blur()
clear()
destroy()
focus()
unmount()
update()
fields.create(type, options)
var card = fields.create('card');
This method creates an instance of a specific Smart Field. It takes the type
of Smart Fields to create as well as an options
object.
Smart Fields Types
Type | Description |
---|---|
card | A flexible single-line input that collects cardNumber, cardExpiry and cardCvc. |
pan | The card‘s number. Use alongside the expiration and cvv Smart Fields. |
expiration | The card‘s expiration date. Use alongside the pan and cvv Smart Fields. |
cvv | The card‘s CVC number. Use alongside the pan and expiration Smart Fields. |
cvv-only | Use this Smart Field if you are only going to tokenize the CVV. |
Examples Checkout
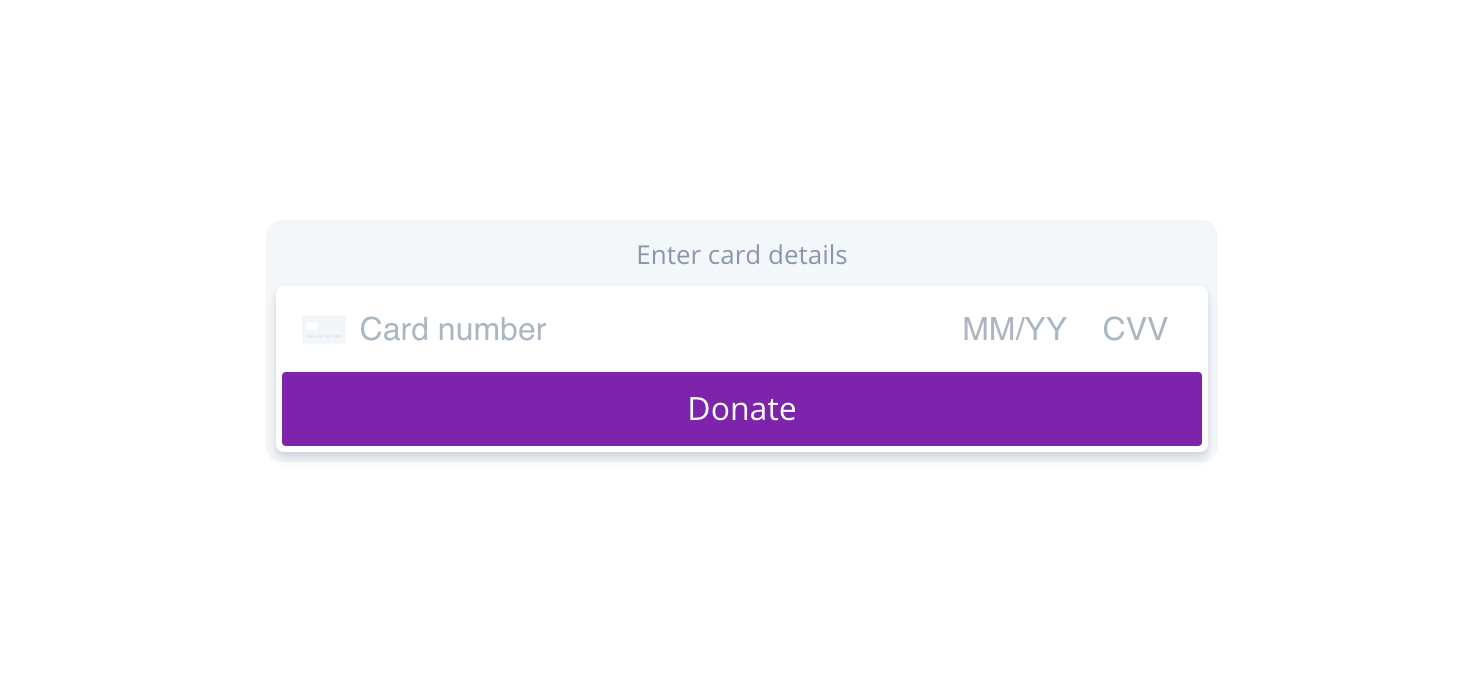
Example Checkout with card
Smart Field
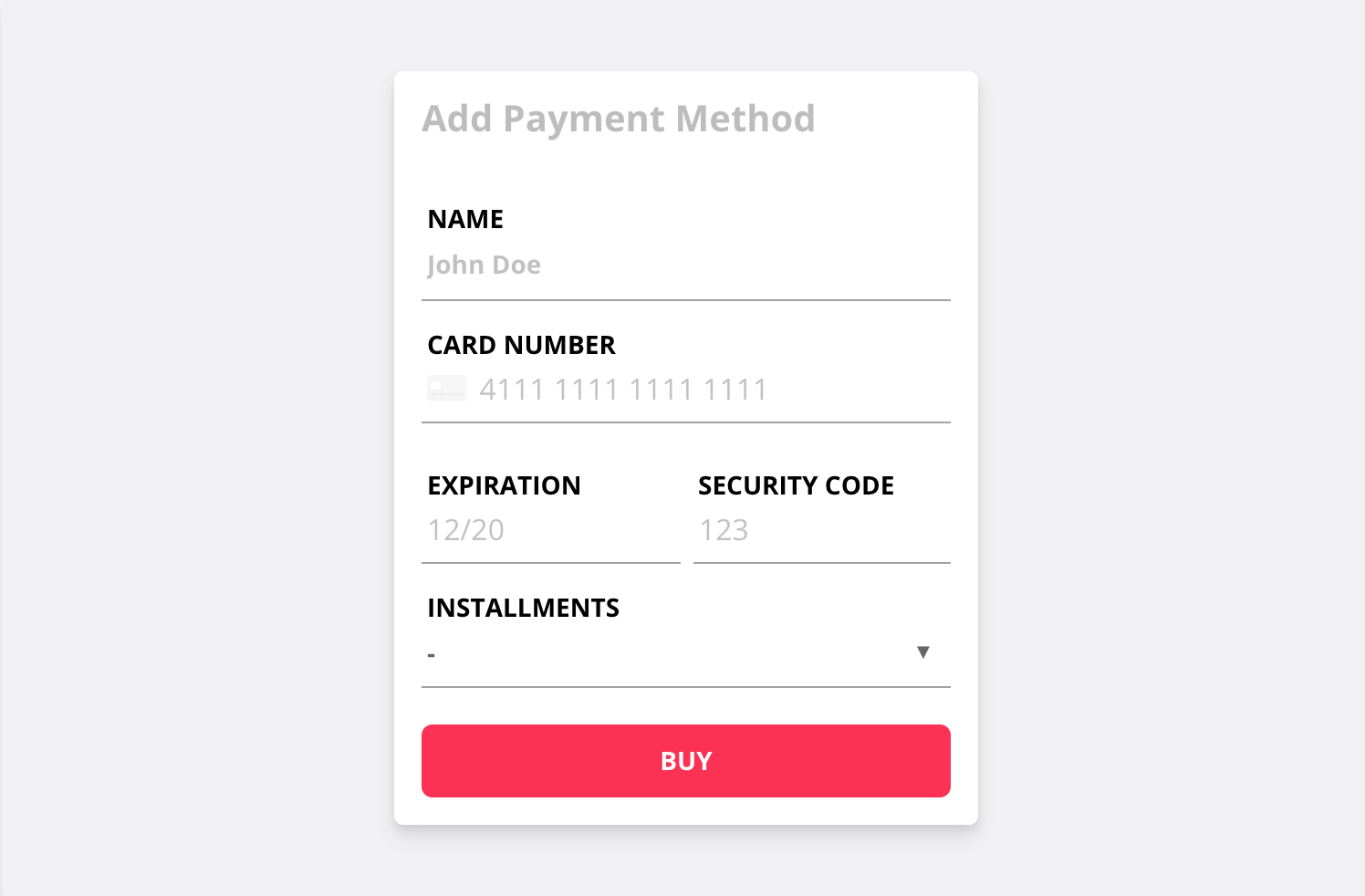
Example Checkout with pan
, expiration
, and cvv
Smart Fields
Field Options
All Smart Fields accept a common set of options, and then some Field-specific options.
Common options
Option | Type | Description |
---|---|---|
placeholder | String or Object | Set custom placeholder for Field. For number , expiration or cvv Fields this option is a string with the corresponding placeholder value, if the Field is a card this option is an object with the corresponding placeholder value for each input:{ cvv: "cvv placeholder", number: "number placeholder", expiration: "expiration placeholder" } |
classes | Object (Optional) | Set custom class names on the container DOM element when the dLocal Field is in a particular state. - base - The base class applied to the container.Defaults to DlocalField. - complete - The class name to apply when the Field is complete.Defaults to DlocalField--complete. - empty - The class name to apply when the Field is empty.Defaults to DlocalField--empty. - focus - The class name to apply when the Field is focused.Defaults to DlocalField--focus. - invalid - The class name to apply when the Field is invalid.Defaults to DlocalField--invalid. - webkitAutofill - The class name to apply when the Field has its value autofilled by the browser (only on Chrome and Safari).Defaults to DlocalField--autofilled. |
style | Object (Optional) | Customize appearance using CSS properties. Style is specified as an object for any of the variants below. - base , base style—all other variants inherit from this style. - complete , applied when the Field has valid input.- empty , applied when the Field has no customer input.- invalid , applied when the Field has invalid input. - autofilled , applied when the Field is autofilled.For each of the above, the properties below can be customized. - color - fontFamily - fontSize - fontSmoothing - fontStyle - fontVariant - iconColor - lineHeight , to avoid cursors being rendered inconsistently across browsers, consider using a padding on the Field's container instead.- letterSpacing - textDecoration - textShadow - textTransform The following pseudo-classes and pseudo-elements can also be styled with the above properties, as a nested object inside the variant. - :hover - :focus - ::placeholder - ::selection - :disabled |
Options available exclusively to the card or number Field
Option | Type | Description |
---|---|---|
iconStyle | String (Optional) | Appearance of the icon in the Field. Either 'solid' or 'default' . |
hideIcon | Boolean (Optional) | Hides the icon in the Field. Default is false . |
Options available exclusively to the CVV Field
Option | Type | Description |
---|---|---|
maskInput | Boolean (Optional) | Masks the CVV input. |
field.mount(domElement)
You need to create a container DOM element to mount a Smart Field. If the container DOM element has a label, the Field is automatically focused when its label is clicked. There are two ways to do this:
- Mount the instance within a
<label>
.
<label>Card
<div id="card-field"></div>
</label>
- Create a
<label>
with afor
attribute, referencing the ID of your container.
<label for="card-field">Card</label>
<div id="card-field"></div>
The field.mount()
method attaches your Field to the DOM. field.mount()
accepts either a CSS Selector (e.g., '#card-field'
) or a DOM element.
cardField.mount('#card-field');
field.on(event, handler)
The only way to communicate with your Smart Field is by listening to an event
. Fields might emit any of the events below. All events have a payload object that has an fieldType
property with the type of the Field that emitted the event.
Event | Description |
---|---|
blur | Triggered when any of the Fields elements loses focus. The event payload always contains certain keys: - empty - Boolean - true if the value is empty.- complete - Boolean -true if the value is well-formed and complete:* complete can be used to progressively disclose the next parts of your form or to enable form submission.* complete is not an indicator of whether a customer is done with their input—it only indicates that the Field contains a complete, well-formed value. - error - The current validation error, if any. Comprised of message , code , and type set to validation_error .- brand - String - The detected card brand, if any.- element - String - The name of the field that triggered the event: 'number' , 'expiration' , 'cvv' .- hasFocus - Boolean - true if any of the Fields elements has focus (always false in pan , expiration and cvv Fields).- autofilled - Boolean - true if any of the Fields is autofilled. |
focus | Triggered when any of the Fields elements gains focus. The event payload always contains certain keys: - empty - Boolean - true if the value is empty.- complete - Boolean -true if the value is well-formed and complete:* complete can be used to progressively disclose the next parts of your form or to enable form submission.* complete is not an indicator of whether a customer is done with their input—it only indicates that the Field contains a complete, well-formed value. - error - The current validation error, if any. Comprised of message , code , and type set to validation_error .- brand - String - The detected card brand, if any.- element - String - The name of the field that triggered the event: 'number' , 'expiration' , 'cvv' .- autofilled - Boolean - true if any of the Fields is autofilled. |
error | Triggered when a client-side validation error is detected. The event payload always contains error key which contains the current validation error. Comprised of: message , code and type , set to validation_error . |
complete | Triggered when the Field changes its complete status. The event payload always contains complete - Boolean - key, which is true when the Field is complete and well-formed, and false otherwise.Important Note: The complete event will only be triggered for cards with BINs identified by Smart Field's BIN detector. Some BINs might not yet be supported by the BIN detector so this event might not be triggered for those. Therefore, you should not expect this event for all cards. |
empty | Triggered when the Field changes its empty status. The event payload always contains empty - Boolean - key, which is true when the Field is empty, and false otherwise. |
ready | Triggered when the Field is mounted and loaded in the DOM. |
change | Triggered when any of the following values changes on the Field. The event payload always contains certain keys, in addition to some Field-specific keys. - empty - Boolean - true if the value is empty.- complete - Boolean -true if the value is well-formed and complete:* complete can be used to progressively disclose the next parts of your form or to enable form submission.* complete is not an indicator of whether a customer is done with their input—it only indicates that the Field contains a complete, well-formed value. - error - The current validation error, if any. Comprised of message , code , and type set to validation_error .- brand - The detected card brand, if any.- autofilled - Boolean - true if any of the Fields is autofilled. |
brand | Triggered when the Field detects a change in the card brand. This event can only be listened in number and card Smart Fields (it won't work in cvv and expiration Fields). The event payload always contains brand - String - key, which has the name of the detected brand if any, null otherwise.If using installments, it is highly recommended that you include the createInstallmentsPlan on the brand event. This is because the installment plan only depends on the amount and card brand. |
autofilled | Triggered when the Field detects a change in it's autofilled status. The event payload always contains autofilled - Boolean - key, which is true if the field is autofilled. |
Input validation
Smart Fields validates customer input as it is typed. To help your customers catch mistakes, listen to change
events on the Field and display any reported errors:
card.addEventListener('change', function(event) {
var displayError = document.getElementById('card-errors');
if (event.error) {
displayError.textContent = event.error.message;
} else {
displayError.textContent = '';
}
});
Other Methods
Method | Description |
---|---|
blur() | Blurs the Field. |
clear() | Clears the value(s) of the Field. |
destroy() | Removes the Field from the DOM and destroys it. Note: a destroyed Field cannot be re-activated or re-mounted to the DOM. |
focus() | Focuses the Field. In a card Field it will focus in the number field. |
unmount() | Unmounts the Field from the DOM. Call field.mount() to re-attach it to the DOM. |
update(options) | Updates the options the Field was initialized with. Updates are merged into the existing configuration. Accepts the same options as fields.create(). |
The styles of a Smart Field can be dynamically changed using update()
. This method can be used to simulate CSS media queries that automatically adjust the size of Fields when viewed on different devices.
window.addEventListener('resize', function(event) {
if (window.innerWidth <= 320) {
card.update({style: {base: {fontSize: '13px'}}});
} else {
card.update({style: {base: {fontSize: '16px'}}});
}
});
var previousBrand;
card.on('change', function(event) {
if (event.brand !== previousBrand && event.brand === 'mastercard') {
card.update({style: {base: {color: 'orange'}}});
previousBrand = event.brand;
}
});