Configure initial settings
Learn how to configure the initial settings in order to access the production platform.
Integration settings
To go live, you need to provide the following information in the Integration section:
- Server's IP addresses for whitelisting. You may specify multiple individual IP addresses or IP address ranges.
- Endpoint URLs for return and notification messages from dLocal.
IP whitelist
Add the IP addresses you want to whitelist:
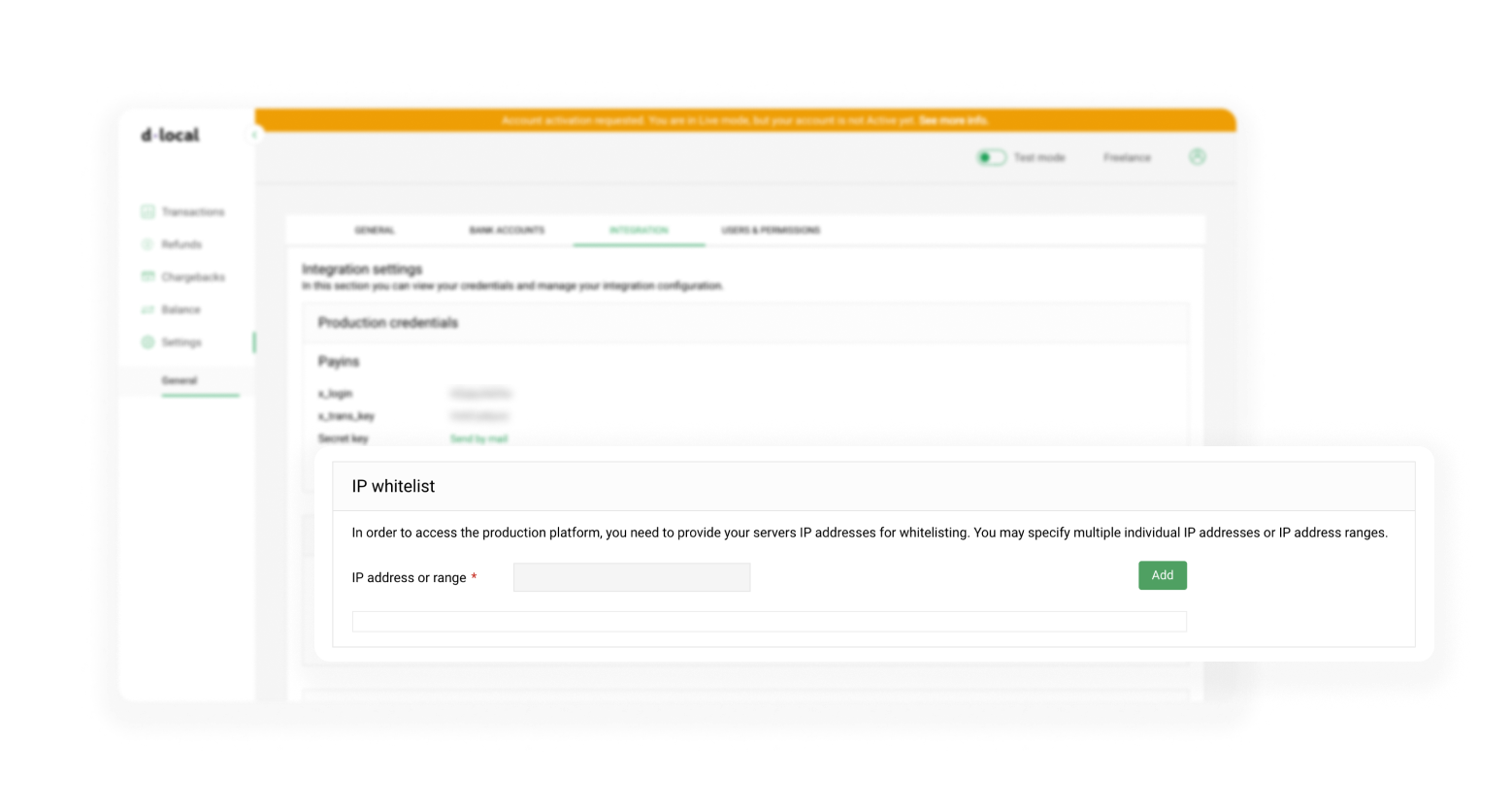
Mutual TLS Certificates
If you can not provide one or more static IPs to whitelist due to infrastructure or network limitations, dLocal API has an alternative subdomain to authenticate your server using certificate-based authentication.
Before starting this process, coordinate it with the Technical Account Manager assigned to you for details.
Requirements
The following steps are required to use this method:
- Purchase a certificate for client authentication to a trusted Certificate Authority. Each certificate authority will have its own methods of delivering the signed certificate but none should ever have contact with the private key. The safest way is when you generate the key pair and create a signing request and send this certificate request to the Certificate authority.
- Once you have the certificate signed by the certificate authority, share that certificate, with the certification path (issuer, subCA, root). dLocal will allow this certificate to be used for authentication.
- Now you have to implement certificate-based authentication in your HTTP client application. This will depend on the programming language your client is coded in (Java, Python, PHP, Ruby, NodeJS), the framework used, and how you safeguard the private key and the certificate.
Implementation examples
package org.example;
import org.apache.http.Header;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.conn.ssl.SSLConnectionSocketFactory;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.ssl.SSLContexts;
import javax.net.ssl.SSLContext;
import java.io.*;
import java.security.*;
import java.security.cert.CertificateException;
public class Main {
public static void main(String[] args) throws KeyStoreException, CertificateException, IOException, NoSuchAlgorithmException, UnrecoverableKeyException, KeyManagementException, InvalidKeyException {
String aliasStore = "testalias", pass = "1234567890"; //here the certificate and trusted store have the same password; it should be different for security reasons
KeyStore clientCertificateStore = KeyStore.getInstance("jks");
FileInputStream clientCertificateFile = new FileInputStream(new File("./src/main/resources/identity.jks"));
clientCertificateStore.load(clientCertificateFile, pass.toCharArray());
KeyStore trustedCAsStore = KeyStore.getInstance("jks");
FileInputStream trustedCAsFile = new FileInputStream(new File("./src/main/resources/truststore.jks"));
trustedCAsStore.load(trustedCAsFile, pass.toCharArray());
SSLContext sslContext = SSLContexts.custom()
.loadKeyMaterial(clientCertificateStore, pass.toCharArray(), (aliases, socket) -> aliasStore)
.loadTrustMaterial(trustedCAsStore, null)
.build();
SSLConnectionSocketFactory sslConnectionSocketFactory = new SSLConnectionSocketFactory(sslContext,
new String[]{"TLSv1.2"},
null,
SSLConnectionSocketFactory.getDefaultHostnameVerifier());
CloseableHttpClient client = HttpClients.custom()
.setSSLSocketFactory(sslConnectionSocketFactory)
.build();
String x_login = "1955gdod";
String x_trans = "j81xh5";
String date = "2022-11-24T15:42:57.130Z";
String auth_signature = Signature.getSignature("", date, x_login);
HttpGet get = new HttpGet("https://sandbox-cert.dlocal.com/payments-methods?country=CO");
get.setHeader("Accept", "application/json");
get.setHeader("Content-type", "application/json");
get.setHeader("User-Agent", "HTTPClient");
get.setHeader("Accept", "*/*");
get.setHeader("Accept-Encoding", "gzip, deflate, br");
get.setHeader("Connection", "keep-alive");
get.setHeader("X-Date", date);
get.setHeader("X-Login", x_login);
get.setHeader("X-Trans-Key", x_trans);
get.setHeader("X-Version", "2.1");
get.setHeader("Authorization", "V2-HMAC-SHA256, Signature: " + auth_signature);
HttpResponse response = client.execute(get);
}
}
import urllib3
from Signature import Signature
if __name__ == '__main__':
http = urllib3.PoolManager(
cert_file='./testseba.cer',
cert_reqs='CERT_REQUIRED',
key_file='./testseba.pkcs8',
key_password='12345678')
x_login = '1955gdod'
x_trans = 'j81xh5'
date = '2022-11-24T15:42:57.130Z'
auth_signature= Signature.getSignature("",date, x_login) #your own signature method
r = http.request(
'GET',
'https://sandbox-cert.dlocal.com/payments-methods?country=CO',
headers={
'Accept': 'application/json',
'Content-type': 'application/json',
'User-Agent': 'HTTPClient',
'Accept': '*/*',
'Accept-Encoding': 'gzip, deflate, br',
'Connection': 'keep-alive',
'X-Date': date,
'X-Login': x_login,
'X-Trans-Key': x_trans,
'X-Version': '2.1',
'Authorization': 'V2-HMAC-SHA256, Signature: '+auth_signature,
}
)
print(r.data.decode('utf-8'))
Endpoint configuration
Input the callback URL, the payins notification URL, and the refunds and chargebacks URL:
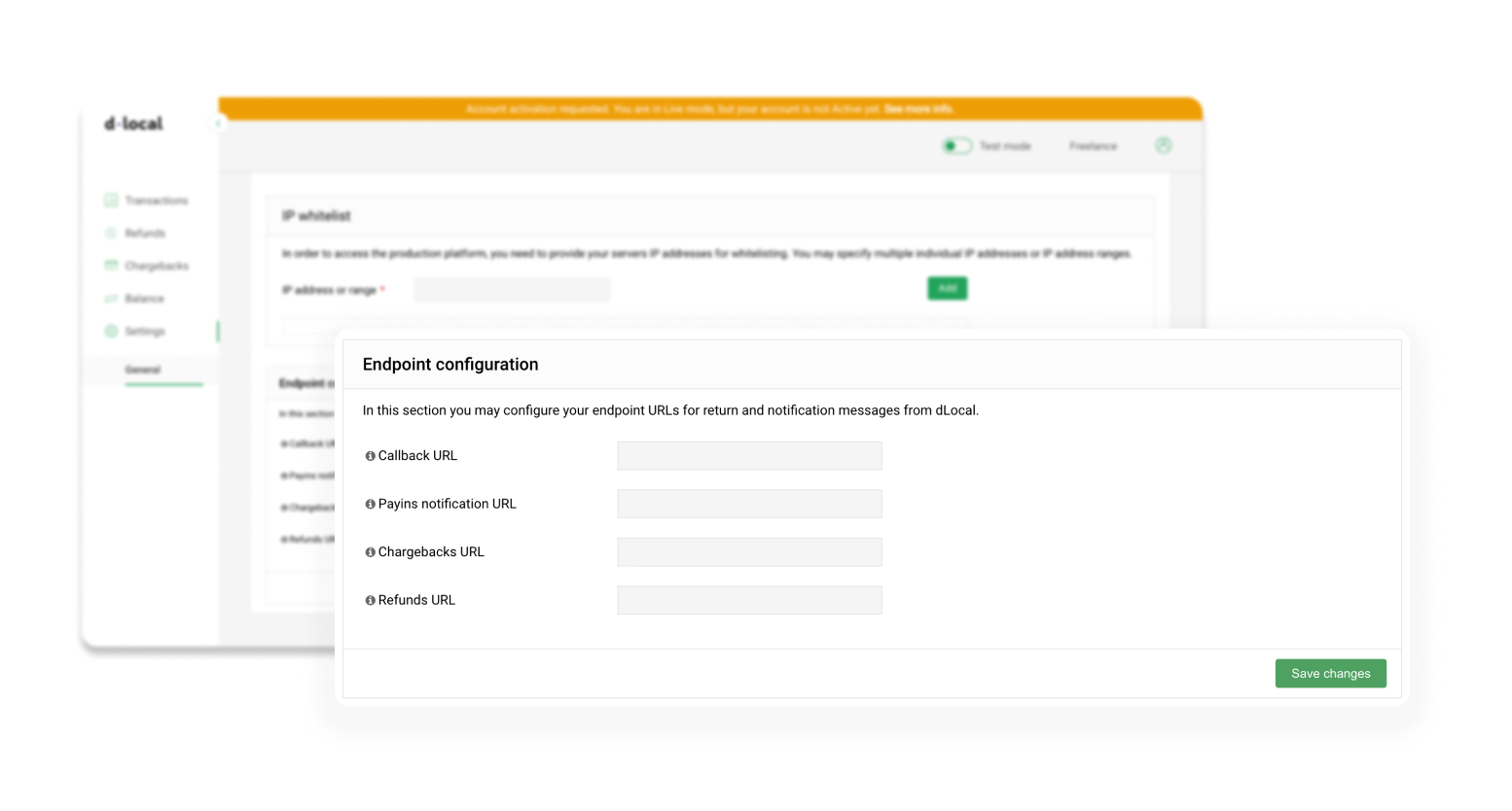
Endpoint details
URL | Description |
---|---|
Callback URL | This is the URL to which the user will be redirected to after the ticket or bank flows ends or the user decides to return. We don’t send notifications here. |
Payins notification URL | Payment status updates are sent to this URL. E.g. when a payment is created and the status is PENDING we will send a notification to this URL once the payment is either approved or rejected/expired. |
Chargebacks URL | We send notifications for chargeback updates to this URL. |
Refunds URL | We send notifications for refund updates to this URL. |
Business-related information
When you are done with the previous settings, and before starting to test in Sandbox, you can start adding some business-related information.
Access Settings > General and input information like company name, main contact information, bank accounts, and more.
Good practices
Add as much information as you can. This will make communications in the future much smoother.
User permissions
You can also start giving access to new users through Settings > Users & Permissions. Input the user email and set the level of access you want to assign them.
Updated 28 days ago
Ready to go live? Enable Live mode to start processing real payments with production credentials.